Cart Integration
How to integrate the Short Video with your native shopping cart
Cart Integration Scope
The cart integration with Short Video is designed to work seamlessly with products created and managed through our CMS dashboard, utilizing the "Magento System" option. This integration is optimized to provide synchronization and functionality for products within this scope.
Products sourced from other sources or platforms may not be fully supported or synchronized with the Short Video cart. For the best experience, we recommend using the "Magento System" option for product management in your CMS dashboard.
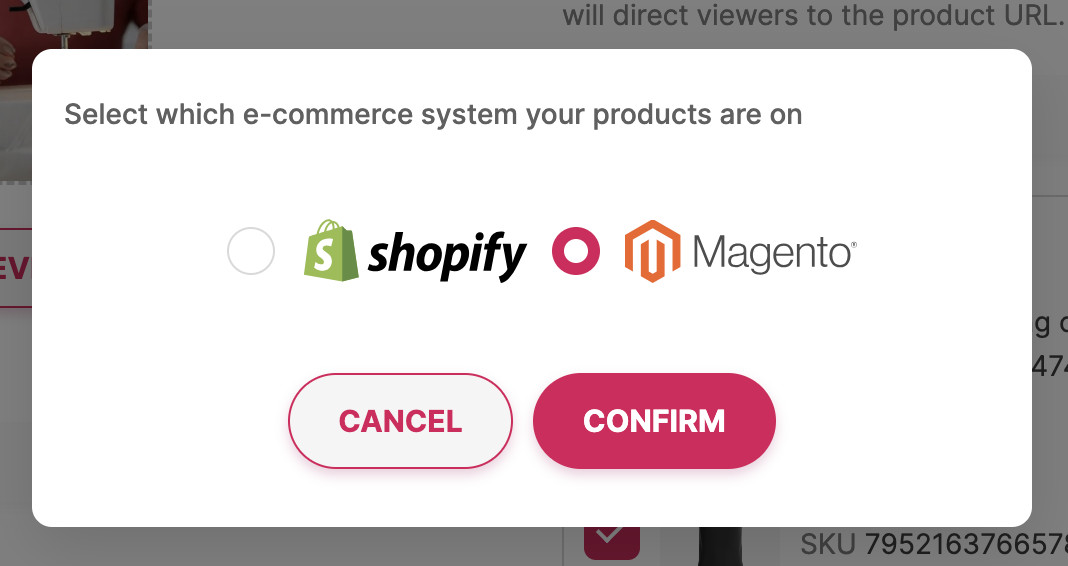
Integrating the Cart with Short Video
Enhancing your live shopping experience with Short Video can be taken a step further by integrating the short video cart with your native shopping cart. This integration enables a seamless shopping journey for your audience. To achieve this, follow the steps below:
Requirements
Before you begin, ensure that your e-commerce app or platform supports the following methods/API endpoints:
- Fetching product details.
- Adding products to the cart.
- Updating items in the cart.
- Checking the cart status (optional).
Implementation
To make use of the short video cart feature, you need to handle some cart-related events. You will find the list of required events and configurations with details and examples below. Required events to be handled
SYNC_PRODUCT
ADD_TO_CART
UPDATE_ITEM_IN_CART
CHECKOUT
SYNC CART STATE
Get the element instance from your embed code
<body>
<story-block-playlist playlist-id="b9181a9c-a793-4b4b-0c46-08da546384f8" video-height="480" loop id="story"></story-block-playlist>
<script>
const storyEl = document.getElementById("story")
</script>
</body>
Providing More Product Data
Event name: SYNC_PRODUCT
Trigger: When the browser render the short video.
In the CMS dashboard, only limited product details are stored, such as the product's title, thumbnail, brand, and a product reference. To enable the cart feature, you can use the event callback to fetch additional product details from your inventory and enrich the products displayed in the show with extra information.
Payload:
Content | Description | Type |
---|---|---|
data (an object that includes the following properties) | playlistId: The ID of the playlist associated with the video. | string |
videoId: The ID of the video. | string | |
productSkus: An array of product SKUs associated with the video. | array | |
callback | To provide your product informations | function |
Example code:
storyEl.eventManager.on('SYNC_PRODUCT', (data, callback) => {
const { playlistId, videoId, productSkus } = data;
// Fetch product details from your inventory based on the productSkus
// Add more product data such as images, variations, and prices
const productDetails = {
// Add your product details here
};
callback(productDetails)
});
In this updated code, we're using the event callback (callback) to provide the enriched product data directly within the event handler. This way, you can fetch the necessary product details and send them to the short video for display when the SYNC_PRODUCT
event is triggered.
Make sure to replace // Add your product details here
with the actual product details you fetch from your inventory.
Handle "Add To Cart" Event
Event name: ADD_TO_CART
Trigger: When a viewer clicks the "Add to Cart" button inside the short video.
In the short video, viewers can add products to the cart. To reflect these actions in your native cart, implement a custom method to add the selected product to the cart within the ADD_TO_CART
event handler.
When callback(true)
, the product will be added to the short video cart.
When callback(false)
, an appropriate error message will appear in the short video
Payload:
Content | Description | Type |
---|---|---|
data (an object that includes the following properties) | playlistId: The ID of the playlist associated with the video. | string |
videoId: The ID of the video. | string | |
itemId: The ID of the item. | string/number | |
quantity: The quantity of the item. | string/number | |
properties: Additional properties or details about the item. | object | |
callback | To inform the short video cart if the process of handling the event was successful or unsuccessful | function |
Example code:
storyEl.eventManager.on('ADD_TO_CART', (data, callback) => {
yourAddToCartMethod(data.sku)
.then(() => {
callback(true); // item successfully added to cart
})
.catch(error => {
if (error.type === 'out-of-stock') {
// Unsuccessful due to 'out of stock'
callback({
success: false,
reason: 'out-of-stock',
});
} else {
// Unsuccessful due to other problems
callback(false);
}
});
});
Handle "Update Cart" Event
Event name: UPDATE_ITEM_IN_CART
Trigger: Whenever the viewer modifies quantity from the short video cart.
In addition to adding items to the cart, a viewer can also increase or decrease the quantity of a previously added item, or even remove the item from the cart.
To reflect this event to you native cart, you will need to use your own method to update the product to the native cart within the UPDATE_ITEM_IN_CART
event handler.
When callback(true)
, the product will be changed to the Short Video cart.
When callback(false)
, an appropriate error message will appear in the short video.
Payload:
Content | Description | Type |
---|---|---|
data (an object that includes the following properties) | playlistId: The ID of the playlist associated with the video. | string |
videoId: The ID of the video. | string | |
id: The ID of the item. | string/number | |
quantity: The quantity of the item. | string/number | |
properties: Additional properties or details about the item. | object | |
callback | To inform the short video cart if the process of handling the event was successful or unsuccessful | function |
Example code:
storyEl.eventManager.on('UPDATE_ITEM_IN_CART', (data, callback) => {
yourUpdateCartMethod({
id: data.id,
quantity: data.quantity,
})
.then(() => {
// cart update was successful
callback(true);
})
.catch(function(error) {
if (error.type === 'out-of-stock') {
callback({
success: false,
reason: 'out-of-stock',
});
} else {
callback(false);
}
});
});
Handle "Go to Checkout" Event
Event name: CHECKOUT
Trigger: When a viewer clicks the "Checkout" button inside the short video cart.
When the viewer is happy with their shopping cart, they can click the Checkout button inside the short video cart.
To handle the checkout process, specify the location of your website's cart within the CHECKOUT
event. This allows viewers to seamlessly transition to the checkout process on your website.
Payload:
Content | Description | Type |
---|---|---|
data (an object that includes the following properties) | playlistId: The ID of the playlist associated with the video. | string |
videoId: The ID of the video. | string |
Example code:
storyEl.eventManager.on('CHECKOUT', (data) => {
yourCheckoutMethod()
});
Handle "Sync the Cart" Event
Event name: SYNC_CART_STATE
Trigger: When the browser render the short video.
In this step, you'll sync the short video cart with the currency, and the contents of your cart to provide a consistent and seamless shopping experience.
Payload:
Content | Description | Type |
---|---|---|
data (an object that includes the following properties) | playlistId: The ID of the playlist associated with the video. | string |
videoId: The ID of the video. | string | |
callback | To provide the current cart content | function |
Example code:
storyEl.eventManager.on('SYNC_CART_STATE', (data, callback) => {
// Fetch the current cart contents from your native cart
const cartContents = await fetchCartContents(); // Implement your own method
callback({
currency: your_currency,
items: cartContents
})
});