Google Tag Manager Integration - LoraStream Analytics Usage Guide
The LoraStreamAnalytics class is a utility for tracking events and analytics related to LORA application. This guide will walk you through how to set up and use this class effectively.
Initialization
To get started with LoraStreamAnalytics, you need to initialize an instance of the class. Here's an example of how to do this:
Step 1: Log in to LORA CMS
- Enter your credentials (username and password) to log in to LORA CMS account.
Step 2: Access the Help Center Page
- Look for the navigation menu or toolbar. Locate and click on the "Help Center" option.
Step 3: Copy the Google Tag Manager Script
- Click on the Copy button under Google Tag Manager to copy Script
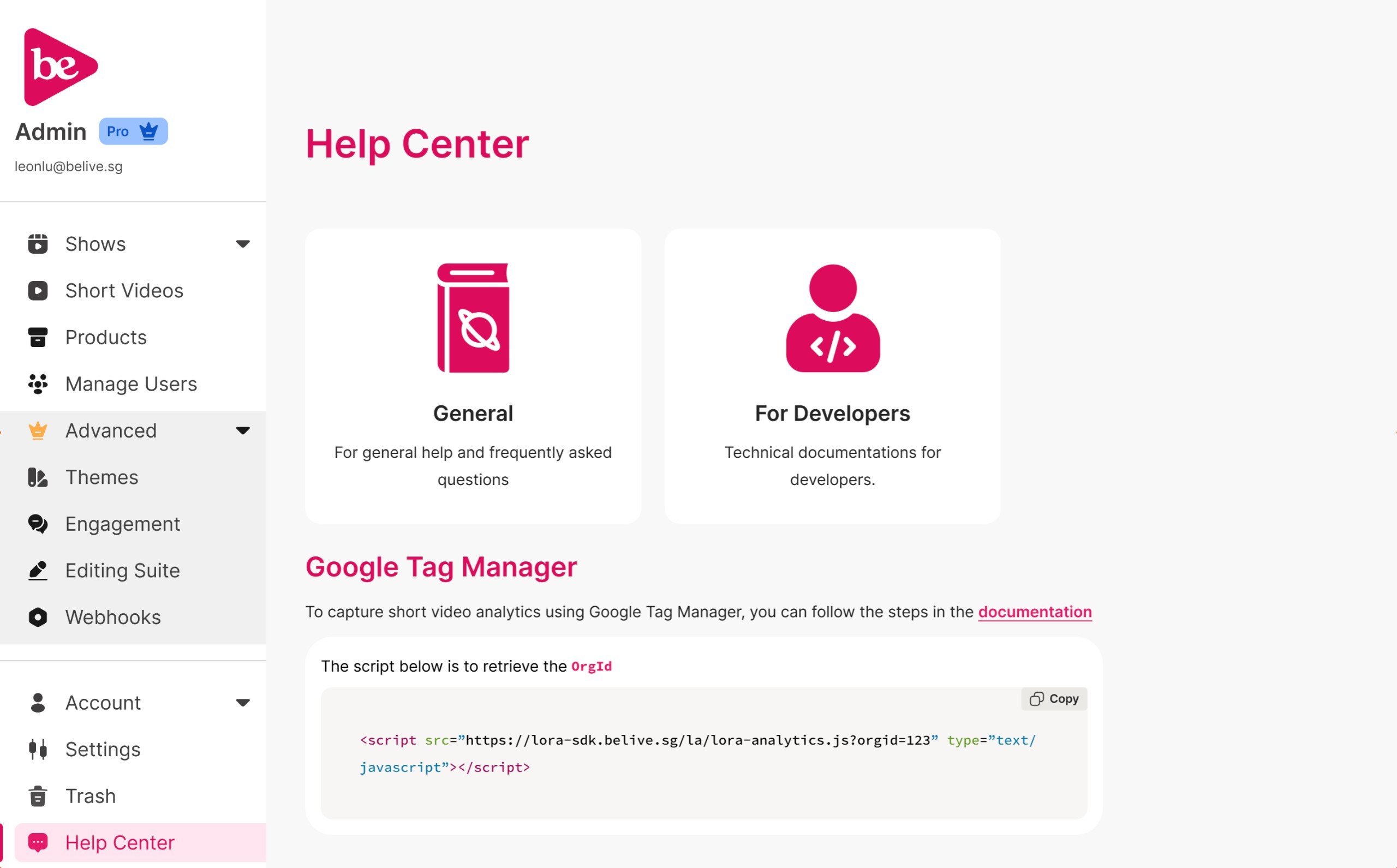
Step 4: Paste the Script in the HTML Code
- Pass the script in the
<head>
tag in your HTML where you want to add the Google Tag Manager script
Example code:
<head>
// Your HTML code .... // Paste the script
<script src="https://lora-sdk.belive.sg/la/lora-analytics.js?orgid=YOUR_ORG_ID" type="text/javascript"></script>
</head>
Step 5: Initial an instance of the class
Example code:
<script>
const liveStreamAnalytics = new window.BeliveShortVideoSdk.LoraStreamAnalytics(YOUR_GTM_CONTAINER_ID); // YOUR_GTM_CONTAINER_ID: if null it will be
LORA GTM
</script>
Tracking Events
The LoraStreamAnalytics class provides several methods for tracking common events:
trackingViewItem
trackingAddToCart
trackingDecreaseFromCart
trackingRemoveFromCart
trackingCheckout
trackingPurchaseSuccess
trackingPurchaseFailed
Tracking view item
trackingViewItem(products, price, currency, data)
Track when a user view an item
Parameters:
Content | Description | Type |
---|---|---|
products | An array of products added to the cart. | array |
price | The total price of the items added. | number |
currency | The currency in which the price is denominated. | string |
custom_data | Additional event-specific data. | object |
Example code:
const products = [
{
item_id: "XYZ789",
item_name: "Product 1",
index: 0,
price: 50,
quantity: 2,
},
{
item_id: "DEF456",
item_name: "Product 2",
index: 1,
price: 100,
quantity: 1,
},
];
const price = 150;
const currency = "USD";
const eventData = {
cart_id: "Cart123",
};
liveStreamAnalytics.trackingViewItem(products, price, currency, eventData);
Tracking add to cart
trackingAddToCart(products, price, currency, data)
Track when a user adds items to their cart with this method.
Parameters:
Content | Description | Type |
---|---|---|
products | An array of products added to the cart. | array |
price | The total price of the items added. | number |
currency | The currency in which the price is denominated. | string |
custom_data | Additional event-specific data. | object |
Example code:
const products = [
{
item_id: "XYZ789",
item_name: "Product 1",
index: 0,
price: 50,
quantity: 2,
},
{
item_id: "DEF456",
item_name: "Product 2",
index: 1,
price: 100,
quantity: 1,
},
];
const price = 150;
const currency = "USD";
const eventData = {
cart_id: "Cart123",
};
liveStreamAnalytics.trackingAddToCart(products, price, currency, eventData);
Tracking decrease item from cart
trackingDecreaseFromCart(products, price, currency, data)
Track when a user decreases items from their cart with this method.
Parameters:
Content | Description | Type |
---|---|---|
products | An array of products added to the cart. | array |
price | The total price of the items added. | number |
currency | The currency in which the price is denominated. | string |
custom_data | Additional event-specific data. | object |
Example code:
const products = [
{
item_id: "XYZ789",
item_name: "Product 1",
index: 0,
price: 50,
quantity: 2,
},
{
item_id: "DEF456",
item_name: "Product 2",
index: 1,
price: 100,
quantity: 1,
},
];
const price = 150;
const currency = "USD";
const eventData = {
cart_id: "Cart123",
};
liveStreamAnalytics.trackingDecreaseFromCart(products, price, currency, eventData);
Tracking remove from cart
trackingRemoveFromCart(products, price, currency, data)
Track when a user remove item from their cart with this method.
Parameters:
Content | Description | Type |
---|---|---|
products | An array of products added to the cart. | array |
price | The total price of the items added. | number |
currency | The currency in which the price is denominated. | string |
custom_data | Additional event-specific data. | object |
Example code:
const products = [
{
item_id: "XYZ789",
item_name: "Product 1",
index: 0,
price: 50,
quantity: 2,
},
{
item_id: "DEF456",
item_name: "Product 2",
index: 1,
price: 100,
quantity: 1,
},
];
const price = 150;
const currency = "USD";
const eventData = {
cart_id: "Cart123",
};
liveStreamAnalytics.trackingRemoveFromCart(products, price, currency, eventData);
Tracking check out
trackingCheckout(products, price, currency, coupon, data)
Track when a user initiates the checkout process.
Parameters:
Content | Description | Type |
---|---|---|
products | An array of products in the cart during checkout. | array |
price | The total price of the items in the cart. | number |
currency | The currency in which the price is denominated. | string |
coupon | The coupon code applied during checkout. | string |
custom_data | Additional event-specific data. | object |
Example code:
const products = [
{
item_id: "XYZ789",
item_name: "Product 1",
index: 0,
price: 50,
quantity: 2,
},
{
item_id: "DEF456",
item_name: "Product 2",
index: 1,
price: 100,
quantity: 1,
},
];
const price = 150;
const currency = "USD";
const coupon = "SAVE10";
const eventData = {
cart_id: "Cart123",
};
liveStreamAnalytics.trackingCheckout(products, price, currency, coupon, eventData);
Tracking purchase success
trackingPurchaseSuccess(products, price, currency, transactionId, coupon, shipping, tax, data)
Track a successful purchase event.
Parameters:
Content | Description | Type |
---|---|---|
products | An array of products included in the purchase. | array |
price | The total purchase price. | number |
currency | The currency in which the price is denominated. | string |
transactionId | The unique identifier of a transaction. | string |
coupon | The coupon code used for the purchase (if applicable). | number | string |
shipping | The shipping info (if applicable). | number | string |
tax | Tax info (if applicable). | number | string |
custom_data | Additional event-specific data. | object |
Example code:
const products = [
{
item_id: "XYZ789",
item_name: "Product 1",
index: 0,
price: 50,
quantity: 2,
},
{
item_id: "DEF456",
item_name: "Product 2",
index: 1,
price: 100,
quantity: 1,
},
];
const price = 150;
const currency = "USD";
const coupon = "SAVE10";
const shipping = 10;
const tax = 12;
const transactionId = "TRSID1234";
const eventData = {
order_id: "Order123",
};
liveStreamAnalytics.trackingPurchaseSuccess(products, price, currency, transactionId, coupon, shipping, tax, eventData);
Tracking purchase failed
trackingPurchaseFailed(products, price, currency, transactionId, coupon, shipping, tax, data)
Track a failed purchase event.
Parameters:
Content | Description | Type |
---|---|---|
products | An array of products in the failed purchase. | array |
price | The total price of the items in the cart during the failed purchase. | number |
currency | The currency in which the price is denominated. | string |
transactionId | The unique identifier of a transaction. | string |
coupon | The coupon code attempted during the failed purchase. | number | string |
shipping | The shipping info (if applicable). | number | string |
tax | Tax info (if applicable). | number | string |
custom_data | Additional event-specific data. | object |
Example code:
const products = [
{
item_id: "XYZ789",
item_name: "Product 1",
index: 0,
price: 50,
quantity: 2,
},
{
item_id: "DEF456",
item_name: "Product 2",
index: 1,
price: 100,
quantity: 1,
},
];
const price = 150;
const currency = "USD";
const coupon = "INVALIDCOUPON";
const shipping = 10;
const tax = 12;
const transactionId = "TRSID1234";
const eventData = {
cart_id: "Cart123",
};
liveStreamAnalytics.trackingPurchaseFailed(products, price, currency, transactionId, coupon, shipping, tax, eventData);