Tracking and Filtering Products in an E-commerce System
Here is example for tracking and filtering products in an E-commerce system
Introduction
In this example, we will explore a simplified process for tracking and filtering products in an e-commerce system. This process allows you to identify products that were viewed or added to the cart during or after a live show or marketing event. We will use JavaScript to demonstrate how to generate tracking URLs, capture and store information, simulate a checkout process, and filter products added from the tracking link.
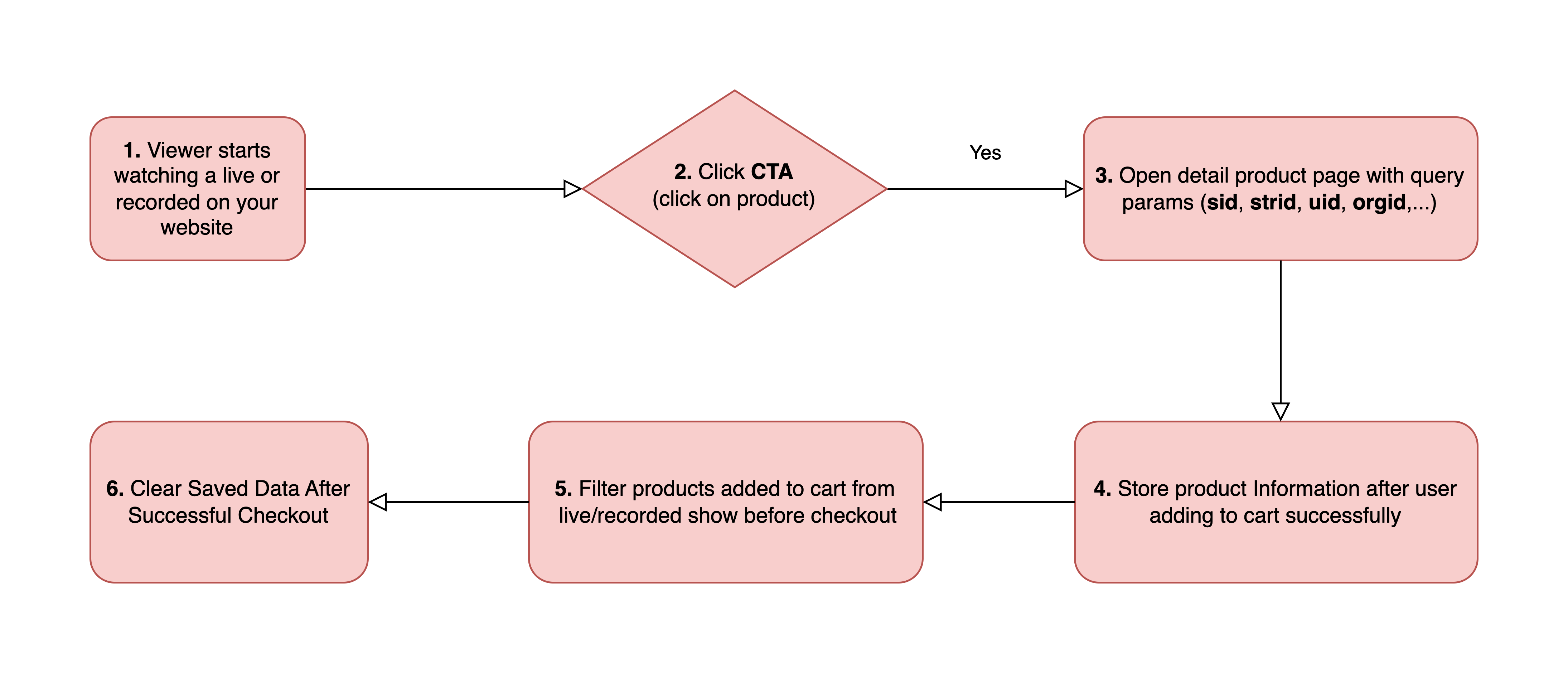
Capture and Store Information
Next, we simulate capturing and storing the tracking URL information when a user arrives at the product page after clicking the CTA or product in the Liveshow. In a real implementation, this data can be conveniently stored locally in cookies, local storage, or any client-side store management system such as Vuex (for Vue.js) or Redux (for React), making it easily accessible and manageable for the user.
Example code:
// Simulate a product object
const product = {
item_id: "XYZ789",
item_name: "Product 1",
index: 0,
price: 50,
quantity: 2,
};
// Simulate adding the product to the cart
yourAddToCartFunction().then(() => {
// If success, capture and store tracking information
captureAndStoreTrackingInfo(product.item_id);
});
function captureAndStoreTrackingInfo(productId) {
// Retrieve previously stored tracking information (if any)
const storedTrackingInfo = JSON.parse(localStorage.getItem("trackingInfo")) || [];
// Store the current productId as part of the tracking information
storedTrackingInfo.push(productId);
// Store the updated tracking information array
localStorage.setItem("trackingInfo", JSON.stringify(storedTrackingInfo));
}
Filter products before checkout
To identify products that were added to the cart from the generated tracking link, we need to filter the products in the cart based on the saved tracking information. In our example, we have simulated a list of products in the cart, and we want to filter this list to include only the products that were added during or after the live show and are associated with the provided tracking information.
Example code:
// Define the cartProducts array with product data in the cart
const cartProducts = [
{
item_id: "XYZ789",
item_name: "Product 1",
index: 0,
price: 50,
quantity: 2,
},
{
item_id: "DEF456",
item_name: "Product 2",
index: 1,
price: 100,
quantity: 1,
},
];
const price = 150;
const currency = "USD";
const coupon = "SAVE10";
const shipping = 10;
const tax = 12;
const transactionId = "TRSID1234";
const eventData = {
order_id: "Order123",
};
// Simulate a successful checkout process
yourCheckoutFunction().then(() => {
// If success, retrieve saved tracking information
const savedTrackingProducts = JSON.parse(localStorage.getItem("trackingInfo")) || [];
// Filter products in the cart based on the saved tracking information
const filteredProducts = cartProducts.filter((product) => {
return savedTrackingProducts.includes(product.item_id);
});
// Call your analytics function with the filtered products and other parameters
liveStreamAnalytics.trackingPurchaseSuccess(filteredProducts, price, currency, transactionId, coupon, shipping, tax, eventData);
});
Clear Saved Data After Successful Checkout
After a successful checkout process, it's important to maintain data cleanliness. This step involves clearing the saved data related to products that were added from the live show. By doing so, you ensure that future tracking and reporting accurately reflect only the most recent interactions, improving the overall tracking and analytics integrity.
Example code:
// Simulate a successful checkout process
yourCheckoutFunction().then(() => {
// Your above code ...
// Clear the saved tracking information
localStorage.removeItem('trackingInfo');
});