Tracking Shopify Cart Integration
Integrate tracking with your Shopify store
Requirements
To utilize this integration for Live Show, you need to have Shopify integration and cart integration.
To begin, initialize the tracking script and create a method to retrieve products from your cart. Then, enrich the cart products with additional information for tracking purposes.
Tracking check out
- Navigate to Online Store > Themes > Actions > Edit code.
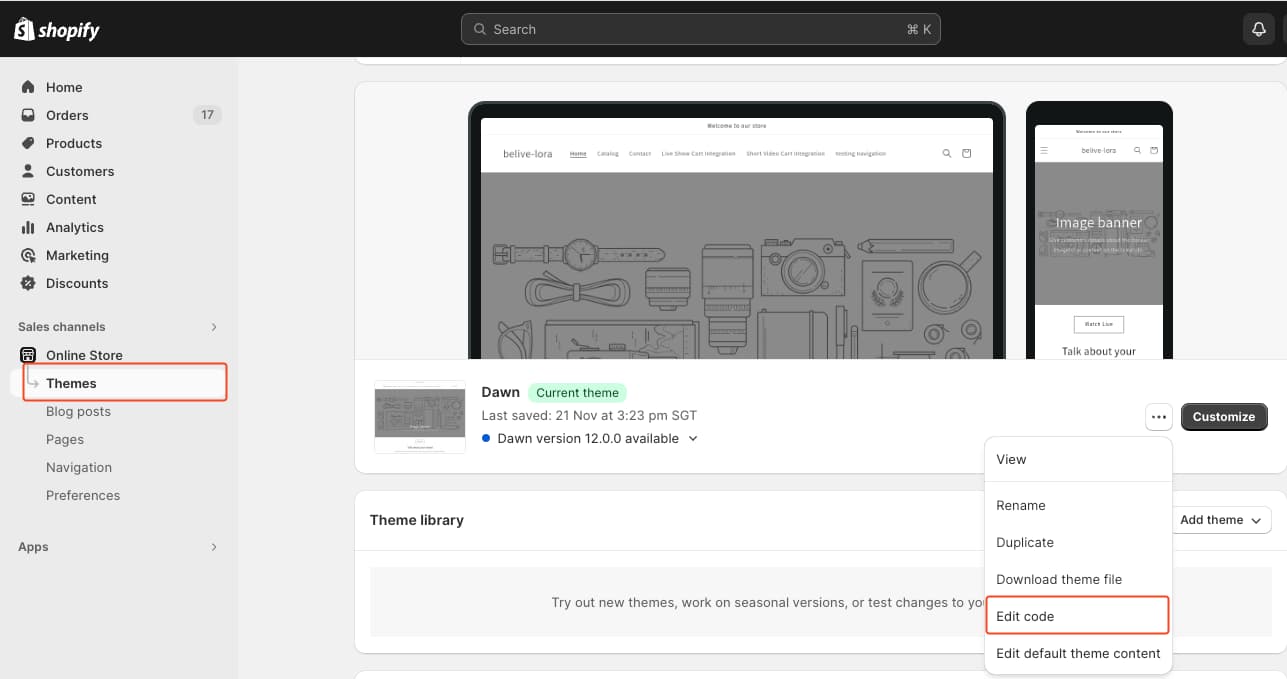
- Embed the tracking script within the
theme.liquid
file, positioned between the HTML<head>
tags. Refer to the guide on embedding the LORA GTM script.
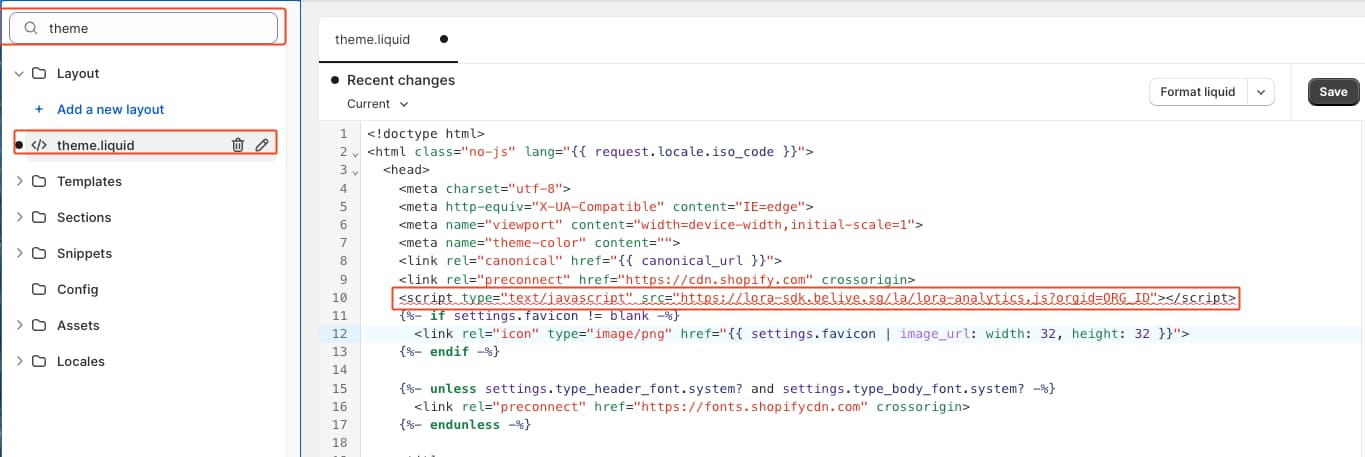
- On your Cart page, switch to the theme editor and add a section named Custom Liquid.
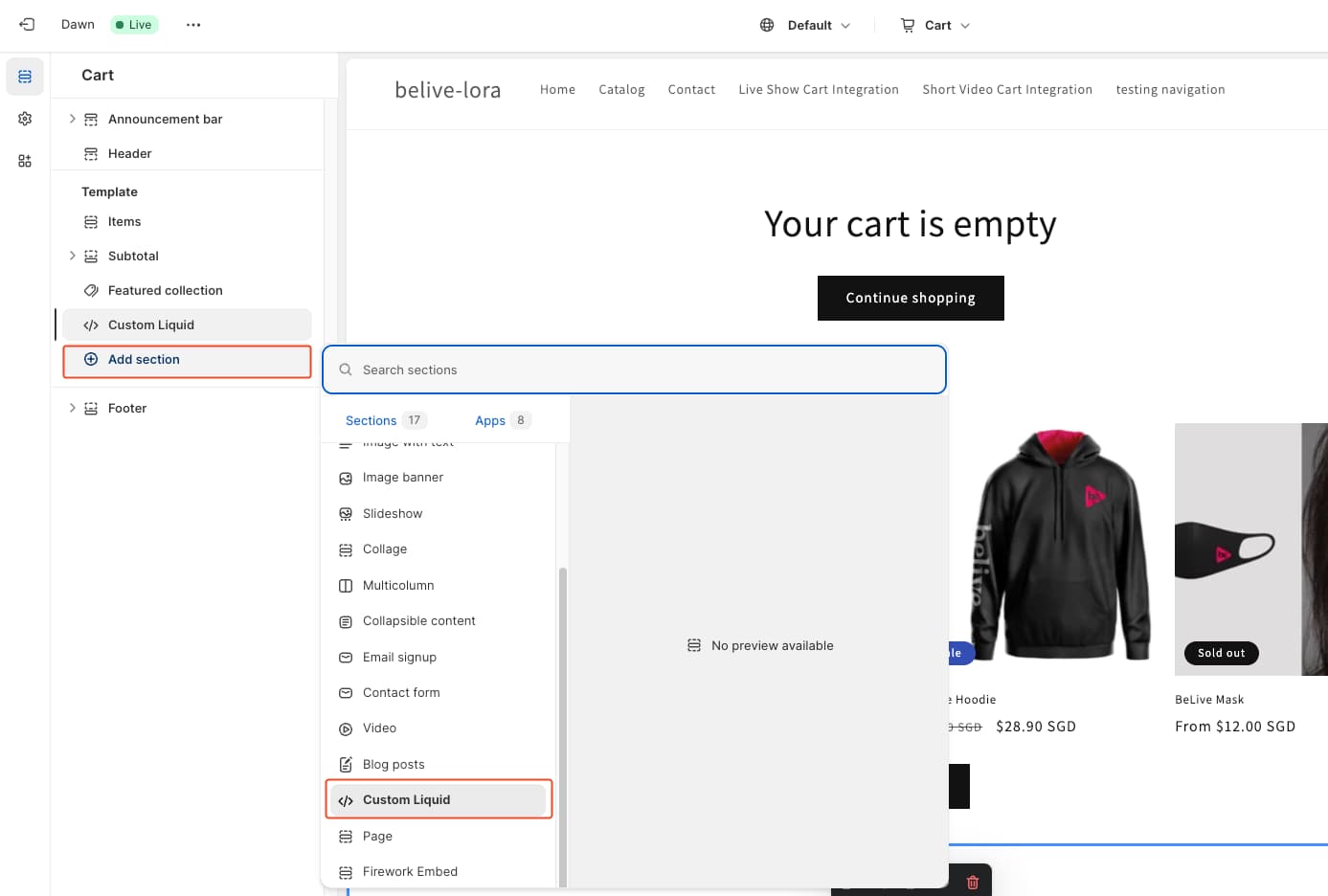
- Paste the embed code into the Custom Liquid section on the right side.
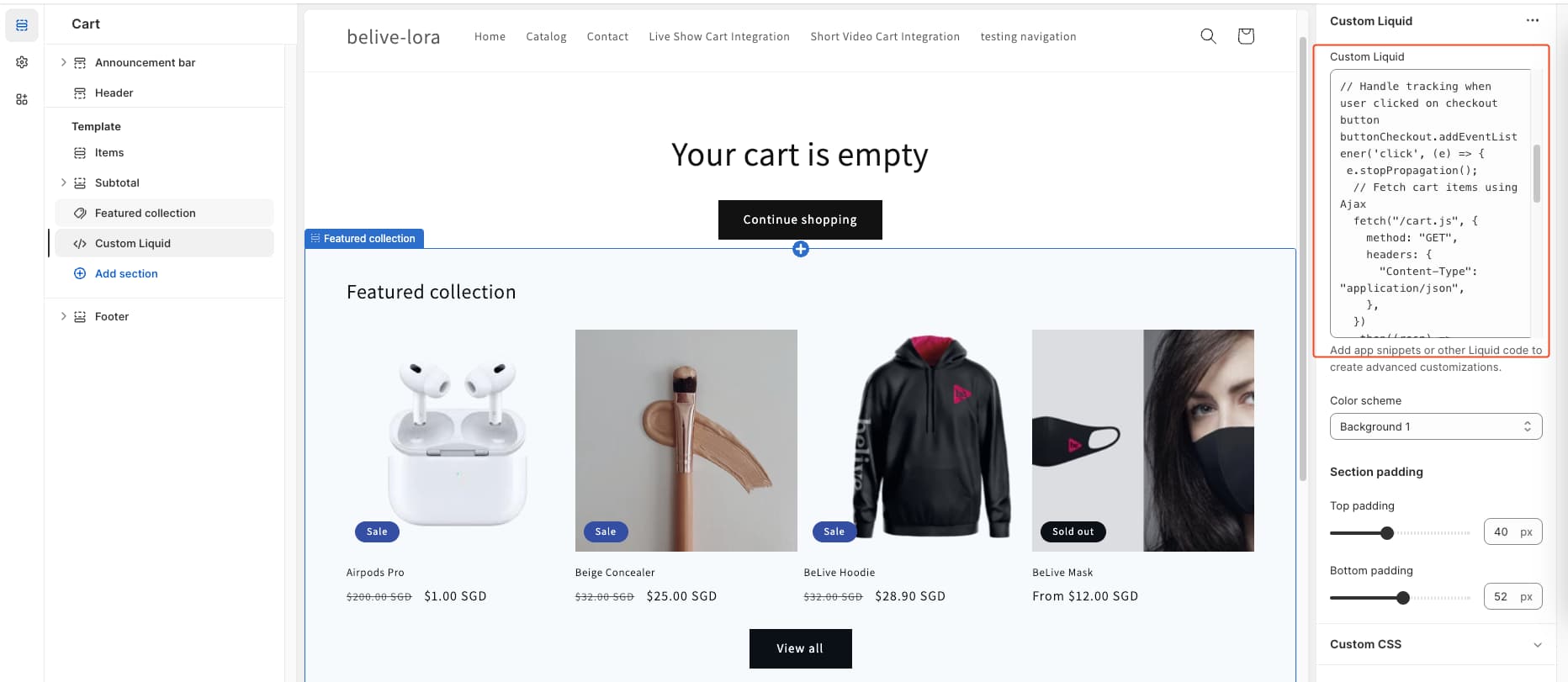
Example code:
<script>
// Initialize your tracking script. You can add your GTM Container ID here
const _myTracking = new window.BeliveShortVideoSdk.LoraStreamAnalytics();
if(!_myTracking) {
console.error('No tracking script initialized');
return;
}
// Retrieve checkout button
const buttonCheckout = document.querySelector('button#checkout');
let cartItems = null;
// Track when the user clicks the checkout button
buttonCheckout.addEventListener('click', () => {
// Fetch cart items using Ajax
fetch("/cart.js", {
method: "GET",
headers: {
"Content-Type": "application/json",
},
})
.then((res) => res.json())
.then((res) => {
cartItems = res
const products = cartItems.items.map(e => ({
...e,
id: e?.product_id.toString(),
item_id: e.product_id,
item_name: e.title,
price: e.price,
quantity: e.quantity
}))
// Send the tracking data to LORA Analytics
_myTracking.trackingCheckout(products, cartItems.total_price, cartItems.currency);
})
.catch((error) => {
// Manage any errors
console.error('Error on tracking checkout', error);
})
});
</script>
- When the user clicks the checkout button, the script will fetch the cart items and send the tracking data to LORA Analytics.
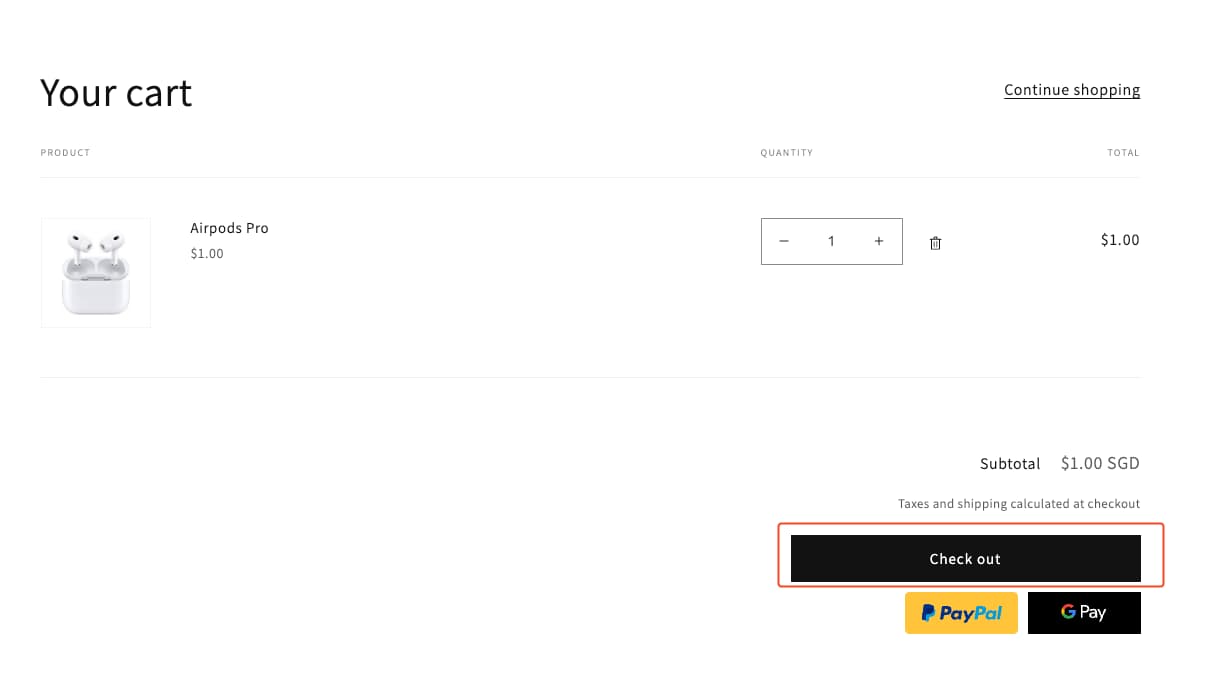
note
If utilizing a custom payment gateway (e.g., Paypal, Gpay), you have the capability to implement the tracking independently.
Tracking purchase success
- Go to the Order Status Page
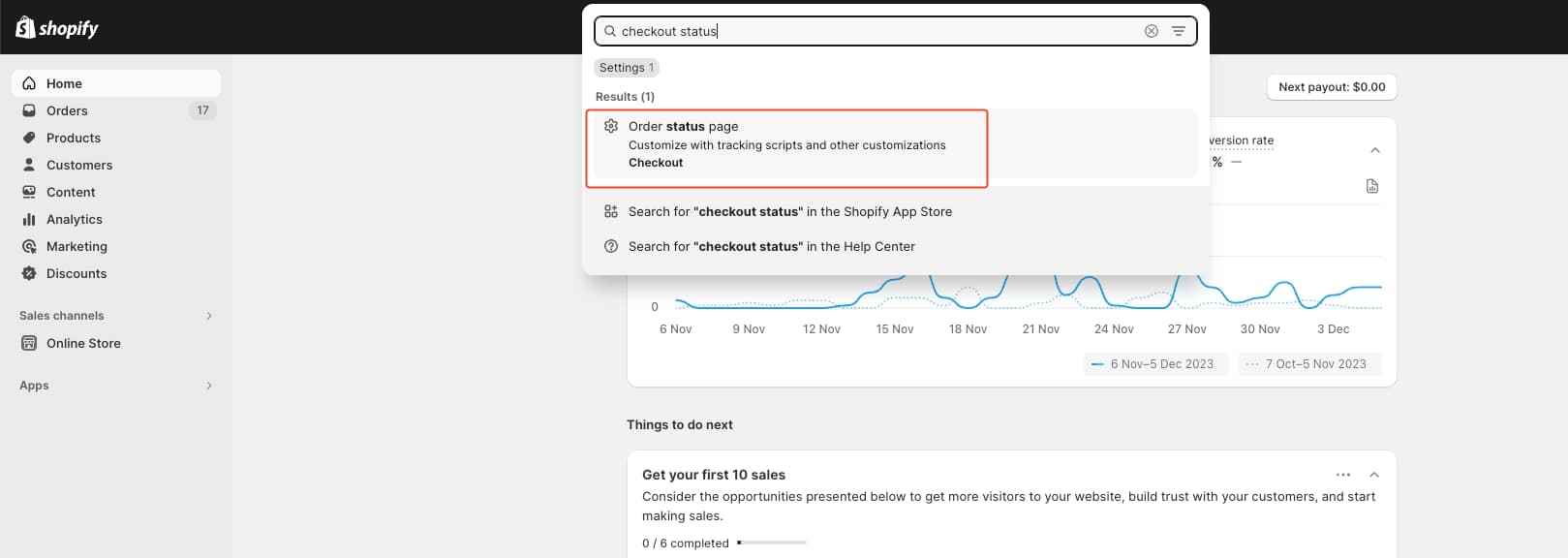
- Embed the tracking script within
Additional scripts
and then Save.
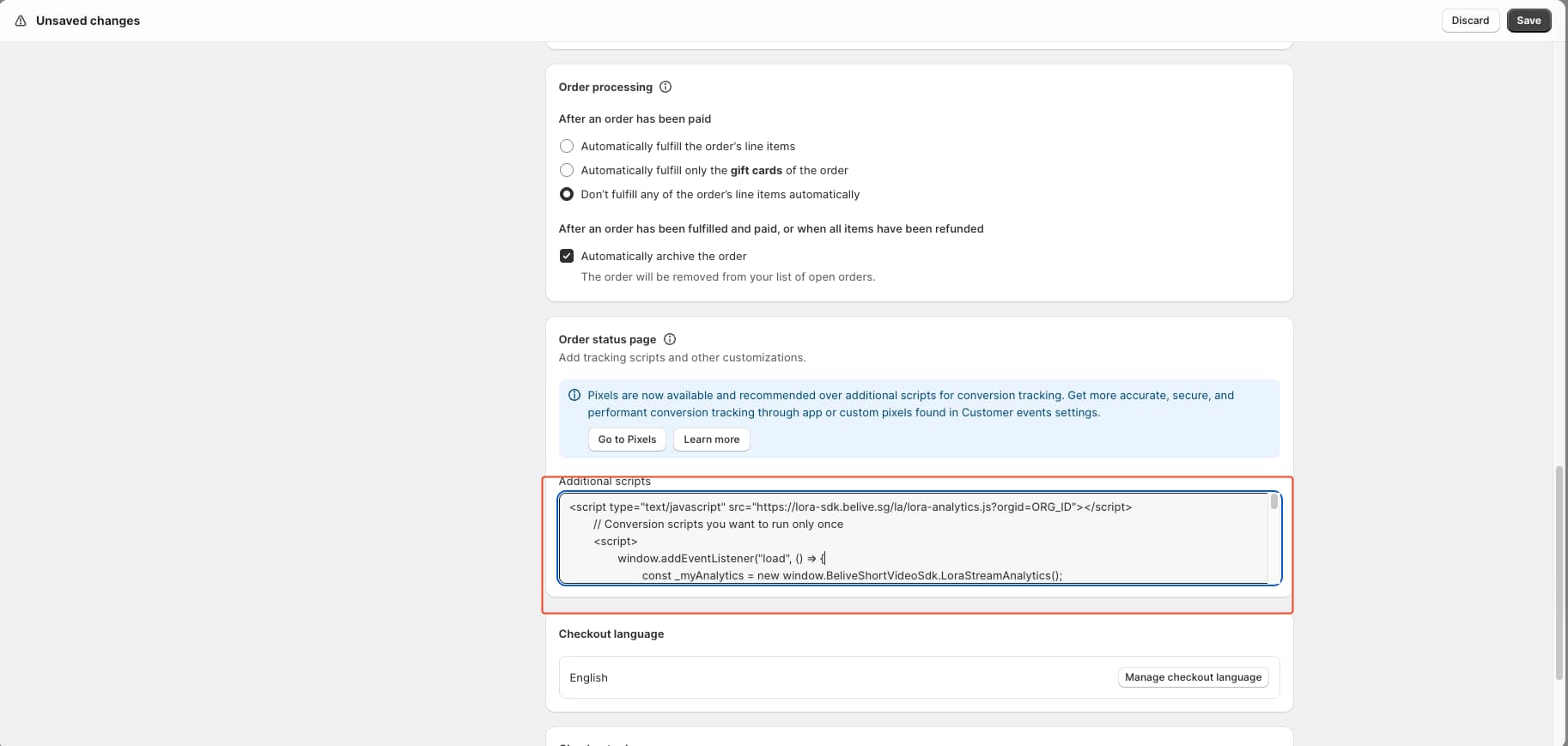
Example code:
// Replace your ORG_ID with your organization ID
<script type="text/javascript" src="https://lora-sdk.belive.sg/la/lora-analytics.js?orgid=ORG_ID"></script>
<script>
{% if first_time_accessed %}
window.addEventListener("load", () => {
const _myAnalytics = new window.BeliveShortVideoSdk.LoraStreamAnalytics();
if(_myAnalytics && !window.dataLayer) {
window.dataLayer = [];
}
if (Shopify && Shopify.checkout && Shopify.Checkout?.isCheckoutOne) {
try {
const currency = Shopify.checkout.currency;
const total = Shopify.checkout.total_price;
const products = Shopify.checkout.line_items.map((item) => {
return {
...item,
item_id: item.product_id,
item_name: item.title,
id: item.product_id.toString(),
name: item.title,
price: item.price,
quantity: item.quantity,
sku: item.sku,
};
});
// Send the tracking data to LORA Analytics
_myAnalytics.trackingPurchaseSuccess([...products], total, currency);
} catch (error) {
console.log('Error on tracking purchase', error);
}
}
})
{% endif %}
</script>
- When the user reaches the
thank you page
after a successful purchase, the script will send the tracking information to LORA Analytics.
Tracking purchase failed
note
If you possess a custom payment failure process or a checkout failure page, you can implement the tracking independently. Refer to this guide on adding the tracking script.